摘要:本教程向您展示如何在 Python 中使用 MySQL BLOB 数据,包括更新和读取BLOB
数据。
authors
表有一个名为photo
的列,其数据类型为 BLOB。我们将从图片文件中读取数据并更新到photo
列。
在 Python 中更新BLOB
数据
首先,开发一个名为read_file()
的函数,用于读取文件并返回文件的内容:
def read_file(filename):
with open(filename, 'rb') as f:
photo = f.read()
return photo
Code language: Python (python)
其次,创建一个名为update_blob()
的新函数,用于更新author_id
指定的作者的照片。
from mysql.connector import MySQLConnection, Error
from python_mysql_dbconfig import read_db_config
def update_blob(author_id, filename):
# read file
data = read_file(filename)
# prepare update query and data
query = "UPDATE authors " \
"SET photo = %s " \
"WHERE id = %s"
args = (data, author_id)
db_config = read_db_config()
try:
conn = MySQLConnection(**db_config)
cursor = conn.cursor()
cursor.execute(query, args)
conn.commit()
except Error as e:
print(e)
finally:
cursor.close()
conn.close()
Code language: Python (python)
让我们详细检查一下代码:
- 首先,我们调用
read_file()
函数从文件中读取数据并返回。 - 其次,我们编写一个UPDATE 语句,更新由
author_id
指定的作者的photo
列。args
变量是一个包含文件数据和author_id
元组。我们将这个变量与query
一起传递给execute()
方法。 - 第三,在
try except
块内,我们连接到数据库,实例化游标,并使用args
执行查询。为了使更改生效,我们调用 MySQLConnection 对象的commit()
方法。 - 第四,我们在
finally
块中关闭游标和数据库连接。
请注意,我们从 MySQL Connector/Python 包中导入了MySQLConnection
和Error
对象,并从我们在上一篇教程中开发的python_mysql_dbconfig
模块中导入了read_db_config()
函数。
让我们测试update_blob()
函数。
def main():
update_blob(144, "pictures\garth_stein.jpg")
if __name__ == '__main__':
main()
Code language: Python (python)
请注意,您可以使用下面的照片并将其放入图片文件夹中进行测试。
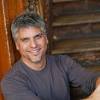
在主函数内,我们调用update_blob()
函数来更新 id 为 144 的作者的照片列。为了验证结果,我们从authors
表中选择数据。
SELECT * FROM authors
WHERE id = 144;
Code language: Python (python)

它按预期工作。
在Python中读取BLOB数据
在此示例中,我们从authors
表中选择BLOB 数据并将其写入文件中。
首先,开发一个write_file()
函数,将二进制数据写入文件:
def write_file(data, filename):
with open(filename, 'wb') as f:
f.write(data)
Code language: Python (python)
其次,创建一个名为read_blob()
的新函数:
def read_blob(author_id, filename):
# select photo column of a specific author
query = "SELECT photo FROM authors WHERE id = %s"
# read database configuration
db_config = read_db_config()
try:
# query blob data form the authors table
conn = MySQLConnection(**db_config)
cursor = conn.cursor()
cursor.execute(query, (author_id,))
photo = cursor.fetchone()[0]
# write blob data into a file
write_file(photo, filename)
except Error as e:
print(e)
finally:
cursor.close()
conn.close()
Code language: Python (python)
read_blob()
函数从authors
表中读取BLOB
数据并将其写入由filename
参数指定的文件中。
代码很简单:
- 首先,编写一个SELECT 语句来检索特定作者的照片。
- 其次,通过调用
read_db_config()
函数获取数据库配置。 - 第三,连接数据库,实例化游标,并执行查询。收到
BLOB
数据后,我们使用write_file()
函数将其写入filename
指定的文件中。 - 最后,关闭游标和数据库连接。
以下代码测试read_blob()
函数:
def main():
read_blob(144,"output\garth_stein.jpg")
if __name__ == '__main__':
main()
Code language: Python (python)
如果打开项目中的输出文件夹并看到其中有图片,则表示您已成功从数据库中读取BLOB
。
在本教程中,我们向您展示了如何使用 MySQL Connector/API 从 Python 更新和读取 MySQL 中的 BLOB 数据。