摘要:在本教程中,我们将逐步向您展示如何使用 Perl DBI 更新 MySQL 表中的数据。
Perl MySQL更新数据步骤
要更新表中的数据,可以使用UPDATE 语句。您可以按照以下步骤使用 DBI 更新 MySQL 表中的数据:
首先,使用connect()
方法连接到 MySQL 数据库。
my $dbh = DBI->connect($dsn,$username,$password, \%attr);
Code language: Perl (perl)
接下来,构造一个UPDATE
语句并将其传递给数据库句柄对象的prepare()
方法以准备要执行的语句。
my $sql = "UPDATE table_name SET col_name = ? WHERE id=?";
my $sth = $dbh->prepare($sql);
Code language: Perl (perl)
然后,如果要将值从 Perl 程序传递到UPDATE
语句,可以在语句中放置占位符 (?),并使用语句句柄对象的bind_param()
方法绑定相应的参数。
$sth->bind_param(1,$value);
$sth->bind_param(2,$id);
Code language: Perl (perl)
之后,调用语句句柄对象的execute()
方法来执行查询。
$sth->execute();
Code language: Perl (perl)
最后,使用disconnect()
方法断开与MySQL数据库的连接。
$dbh->disconnect();
Code language: Perl (perl)
Perl MySQL 更新数据示例
以下示例更新链接 ID 为 2 的 links 表中的数据。在更新数据之前,我们首先检查 links 表:
SELECT * FROM links;
Code language: SQL (Structured Query Language) (sql)
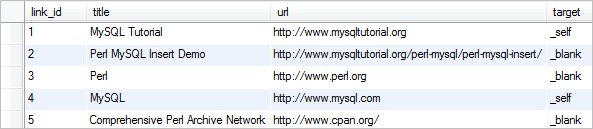
请看下面的程序:
#!/usr/bin/perl
use strict;
use warnings;
use v5.10; # for say() function
use DBI;
# MySQL database configurations
my $dsn = "DBI:mysql:perlmysqldb";
my $username = "root";
my $password = '';
say "Perl MySQL Update Data Demo";
# connect to MySQL database
my %attr = ( PrintError=>0, RaiseError=>1);
my $dbh = DBI->connect($dsn,$username,$password, \%attr);
# update statement
my $sql = "UPDATE links
SET title = ?,
url = ?,
target = ?
WHERE id = ?";
my $sth = $dbh->prepare($sql);
my $id = 2;
my $title = "Perl MySQL Update Data Tutorial";
my $url = "https://www.mysqltutorial.org/perl-mysql/perl-mysql-update/";
my $target = "_self";
# bind the corresponding parameter
$sth->bind_param(1,$title);
$sth->bind_param(2,$url);
$sth->bind_param(3,$target);
$sth->bind_param(4,$id);
# execute the query
$sth->execute();
say "The record has been updated successfully!";
$sth->finish();
# disconnect from the MySQL database
$dbh->disconnect();
Code language: Perl (perl)
该程序的输出是:
Perl MySQL Update Data Demo
The record has been updated successfully!
让我们再次查询links
表以验证更新。
SELECT * FROM links;
Code language: SQL (Structured Query Language) (sql)
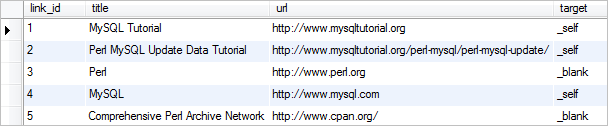
id为2的链接记录已更改成功。
在本教程中,您学习了如何使用 Perl DBI 中的准备语句来更新 MySQL 表中的数据。
本教程有帮助吗?